Let’s start with the basics
Introduced at WWDC 2019, SwiftUI is a declarative UI framework from Apple that enables developers to build native user interfaces for apps running on either iOS, iPadOS, watchOS, tvOS or macOS. Its highly declarative API makes it quite different from Apple’s previous UI frameworks, such as UIKit and AppKit, which in turn often requires us to adopt a somewhat different set of patterns and conventions when starting to use it.
On this Discover page, you’ll find links to lots of articles, tips and other content that all aims to give you a thorough understanding of some of those patterns, as well as how SwiftUI’s built-in suite of APIs and features work.
The SwiftUI layout system
SwiftUI features a brand new layout system that in many ways encourages us to write more dynamic UIs that can scale and adjust according to factors like what device that our code is being run on, what accessibility settings that the user has enabled, and what locale that is currently being used.
What that means in practice is that, similar to when using Auto Layout, we need to write our layout code in a way that makes fewer assumptions about the overall environment that a given view will be rendered in. However, unlike Auto Layout, a SwiftUI layout is determined entirely by the view hierarchy itself, rather than by using dedicated objects like constraints.
Video An introduction to stacks and spacers: two of SwiftUI’s most fundamental layout tools
Article Code along with this three-part guide to SwiftUI’s layout system
Question How to sync the width or height of two SwiftUI views?
Article Using SwiftUI’s frame modifier to resize and align views
Article Backgrounds and overlays
Question When does the order of SwiftUI modifiers matter, and why?
State management
When building our views declaratively, we also need to define our state in a declarative manner as well. Thankfully, SwiftUI ships with a comprehensive suite of state management tools, that range from property wrappers like @State
and @Binding
, to powerful dependency management mechanisms, such as the Environment
API.
Building asynchronous views
Very often when building modern apps, we need to fetch the data for our views asynchronously, for example over the network. While doing that, we probably want to show the user some form of progress view, and we might also want to enable our views to be manually refreshed using the very popular pull-to-refresh mechanism. The following two articles show various techniques for implementing such asynchronous views using SwiftUI:
View styling
Apart from defining layouts and binding various kinds of state to our views, SwiftUI’s modifier system can also be used to apply custom styles to each of the views that we create. Here are a few ways to perform that kind of styling, and some tips on how to encapsulate and share such styles across an application:
Behind the scenes
What better way to learn more about SwiftUI than to hear from some of the people who actually built it? These two podcast episodes feature Josh Shaffer, engineering director with the UIKit and SwiftUI team, and Eliza Block, engineer on Xcode Previews, and contain a ton of interesting behind-the-scenes information on how these developer tools are designed, built and used by Apple.
Interoperability with UIKit and AppKit
At first glance, it might seem like adopting SwiftUI requires us to rewrite our entire user interface using it, but that couldn’t be further from the truth.
In fact, one of SwiftUI’s most powerful features is its UIKit and AppKit interoperability, which both enables us to bring in UIView
and NSView
instances (as well as their view controller equivalents) into the declarative world of SwiftUI, and vice versa.
That gives us a ton of flexibility when it comes to adopting SwiftUI within an existing project, as we can start by building just a single view using it, all while leveraging our existing views and components. The following articles cover various ways to do just that:
Next, a quick message from this week’s sponsor
Swift by Sundell is completely free for everyone, but if you’d like to support my work, then please take just a few minutes to check out the following sponsor, since doing so really helps me out financially.
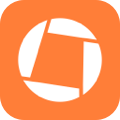
Swift by Sundell is brought to you by the Genius Scan SDK — Add a powerful document scanner to any mobile app, and turn scans into high-quality PDFs with one line of code. Try it today.
The Swift features that power SwiftUI’s API
Next, let’s take a look under the hood of SwiftUI’s very “DSL-like” API. While SwiftUI is a closed-source project that’s privately implemented by Apple, we can get a quite good understanding of how it operates by examining a few key Swift features that its API makes heavy use of. Let’s dive in, shall we?
View architecture
The fact that SwiftUI views are declared using structs and other value types gives us many different options when it comes to what kind of architecture that we’d like to use when building an app’s view layer. The following articles cover some of those options, along with tips and techniques that can be good to keep in mind along the way.
Code structure
Just like when using other UI frameworks, such as UIKit and AppKit, what kind of structure that we use to organize each view’s implementation can have a huge impact on the overall readability and maintainability of our code. Here are a few tips on how maintain a solid structure within each of our SwiftUI views:
Tip Using SwiftUI’s ViewBuilder attribute with functions
Tip Annotating properties with SwiftUI’s ViewBuilder attribute
Tip Creating custom SwiftUI container views
Tip Passing methods as SwiftUI view actions
Article Using multiple computed properties to form a SwiftUI view’s body
Tip SwiftUI extensions using generic type constraints
Customized view rendering
While SwiftUI was definitely designed around making it as easy as possible to build views that are based on system components, it also offers several APIs and features that enable us to create UIs that are rendered in more custom ways. Here are a few examples:
Debugging and developer tools
Next, let’s take a look at how we can improve the overall speed and experience of developing a SwiftUI-based app, both using the built-in tools that ship as part of Xcode, and by creating our own utilities as well.
How is the community using SwiftUI?
SwiftUI is of course a very frequent topic on the Swift by Sundell podcast, with special guests from around the community sharing their tips and experiences of working with SwiftUI in different ways.
Podcast episode Meng To’s top tips on how to build scalable and flexible UIs using SwiftUI
Podcast episode Kaya Thomas on how SwiftUI is a huge step forward in terms of iOS app accessibility
Podcast episode David Smith shares his learnings from deploying SwiftUI in production
Podcast episode How Tobias Due Munk uses SwiftUI to build tools and prototypes
Podcast episode An in-depth discusssion about the SwiftUI layout system with Chris Eidhof
Podcast episode Adam Bell talks about using SwiftUI to visualize audio
Podcast episode Brent Simmons on how SwiftUI marks the start of a new era for Apple
Podcast episode Comparing SwiftUI and Catalyst with Benedikt Terhechte
When is the right time to adopt SwiftUI?
Finally, let’s explore the topic of deciding whether to adopt SwiftUI within a given project, and also how building internal tools and prototypes can provide a great way to get started with SwiftUI, while also minimizing the risks associated with being an early adopter.