This article has been archived, as it was published several years ago, so some of its information might now be outdated. For more recent articles, please visit the main article feed.
How can a SwiftUI Text with mixed styling be created?
Discover page available: SwiftUIWhen building views using SwiftUI, the Text
type can be used to define various strings that are going to be displayed within our UI. However, SwiftUI does not include a built-in equivalent to NSAttributedString
, which is commonly used to style text in various ways when using frameworks like UIKit and AppKit.
So while a Text
value can be styled as a whole — using modifiers like bold()
and italic()
— at first it might seem like we need to place multiple Text
instances into something like an HStack
in order to create a text that has multiple mixed styles:
struct PromotionView: View {
var productName: String
var body: some View {
HStack(spacing: 5) {
Text("Check out")
Text(productName).bold()
Text(",")
Text("it’s amazing!").italic()
}
}
}
However, while the above approach might work initially, it does have a few downsides. Not only do we have to manually manage line breaks, but we also have to decide what exact spacing to use between each text component — which gets quite complicated when we start considering things like dynamic text sizes and accessibility.
Thankfully, there is a much better way to accomplish something like the above, and that’s to simply concatenate multiple Text
values using the +
operator — just like when dealing with normal String
values:
struct PromotionView: View {
var productName: String
var body: some View {
Text("Check out ") +
Text(productName).bold() +
Text(", ") +
Text("it’s amazing!").italic()
}
}
Now, we no longer have to manually manage the layout of our text, and we get complete support for all sorts of dynamic text features for free, all while still being able to render text with mixed styling within our UI.
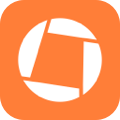
Swift by Sundell is brought to you by the Genius Scan SDK — Add a powerful document scanner to any mobile app, and turn scans into high-quality PDFs with one line of code. Try it today.