This article has been archived, as it was published several years ago, so some of its information might now be outdated. For more recent articles, please visit the main article feed.
Using key paths to create convenience APIs
Basics article available: Map, FlatMap and CompactMapSwift’s key paths can let us build APIs that are both really powerful, but also look really nice and clean at the call site. Here we have an extension that lets us easily group any Sequence
based on an Element
key path:
// This extension will let us group any Sequence (such as an
// Array or a Set), based on a given key path.
extension Sequence {
func grouped<T: Hashable>(by keyPath: KeyPath<Element, T>) -> [T: [Element]] {
// Using key path subscripting syntax, we can dynamically
// access a member of a type based on a key path.
return .init(grouping: self, by: { $0[keyPath: keyPath] })
}
}
func scan(_ string: String, using matchers: [Matcher]) {
// The result is that our call sites become really clean, since
// we can simply use a key path literal to group any sequence.
let matchersByPattern = (
start: matchers.grouped(by: \.pattern.start),
end: matchers.grouped(by: \.pattern.end)
)
...
}
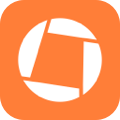
Swift by Sundell is brought to you by the Genius Scan SDK — Add a powerful document scanner to any mobile app, and turn scans into high-quality PDFs with one line of code. Try it today.