This article has been archived, as it was published several years ago, so some of its information might now be outdated. For more recent articles, please visit the main article feed.
Swift 5.7’s new optional unwrapping syntax
Basics article available: OptionalsSwift 5.7 introduces a new, more concise way to unwrap optional values using if let
and guard let
statements. Before, we always had to explicitly name each unwrapped value, for example like this:
class AnimationController {
var animation: Animation?
func update() {
if let animation = animation {
// Perform updates
...
}
}
}
But now, we can simply drop the assignment after our if let
statement, and the Swift compiler will automatically unwrap our optional into a concrete value with the exact same name:
class AnimationController {
var animation: Animation?
func update() {
if let animation {
// Perform updates
...
}
}
}
Neat! The above new syntax also works with guard
statements:
class AnimationController {
var animation: Animation?
func update() {
guard let animation else {
return
}
// Perform updates
...
}
}
It can also be used to unwrap multiple optionals all at once:
struct Message {
var title: String?
var body: String?
var recipient: String?
var isSendable: Bool {
guard let title, let body, let recipient else {
return false
}
return ![title, body, recipient].contains(where: \.isEmpty)
}
}
A small, but very welcome feature. Of course, we still have the option to explicitly name each unwrapped optional if we wish to do so — either for code style reasons, or if we want to give a certain optional a different name when unwrapped.
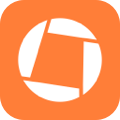
Swift by Sundell is brought to you by the Genius Scan SDK — Add a powerful document scanner to any mobile app, and turn scans into high-quality PDFs with one line of code. Try it today.